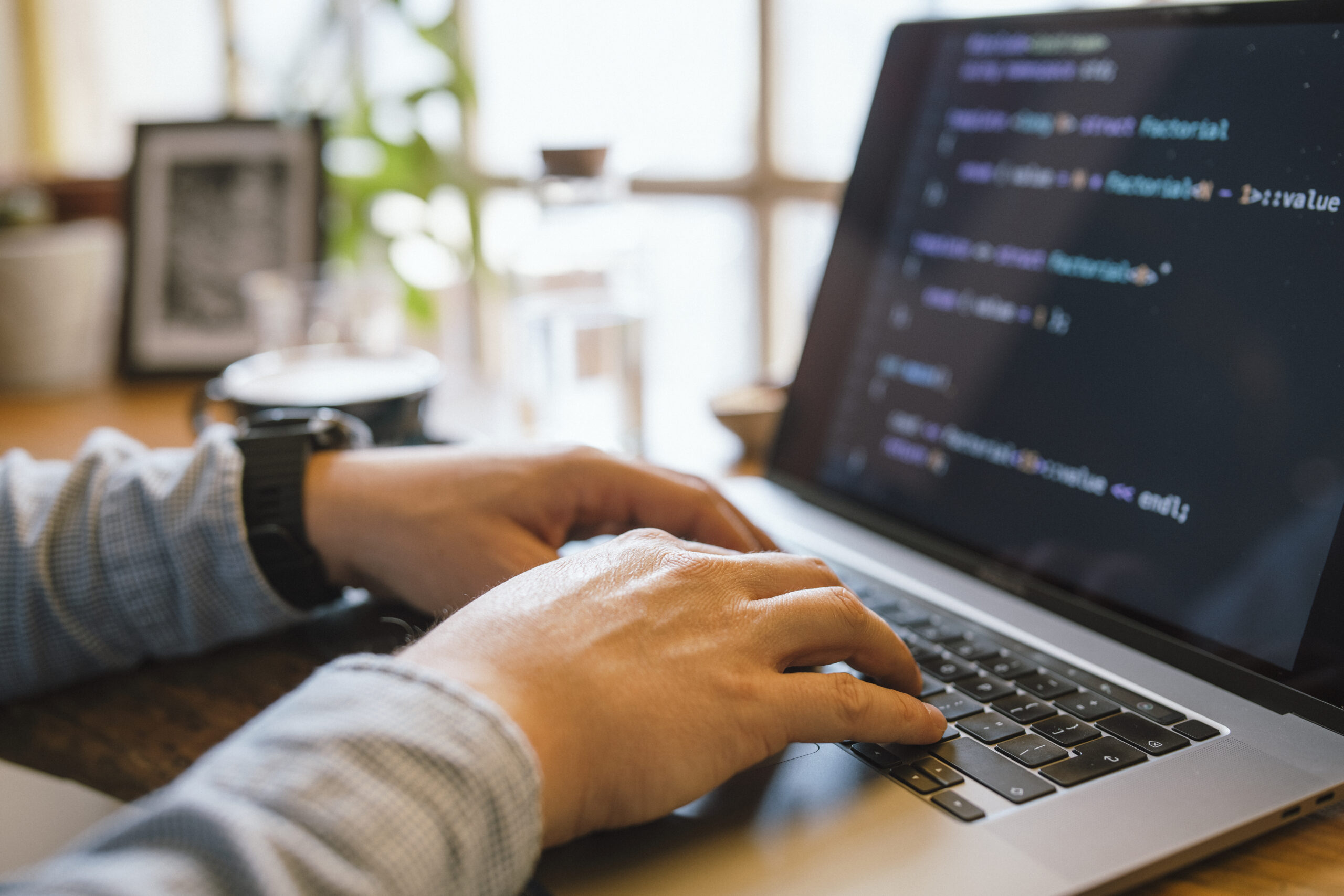
Debugging is one of the most vital — nonetheless frequently disregarded — capabilities in the developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why issues go Completely wrong, and learning to Feel methodically to resolve troubles successfully. Irrespective of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and considerably help your efficiency. Here i will discuss quite a few tactics that can help developers stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies builders can elevate their debugging skills is by mastering the applications they use on a daily basis. When composing code is 1 Section of advancement, understanding how to connect with it properly in the course of execution is equally essential. Fashionable growth environments arrive Geared up with strong debugging capabilities — but numerous builders only scratch the surface area of what these tools can perform.
Consider, for example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, step by code line by line, and in some cases modify code about the fly. When utilized effectively, they Allow you to observe particularly how your code behaves throughout execution, which happens to be invaluable for monitoring down elusive bugs.
Browser developer tools, such as Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep track of network requests, perspective serious-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert frustrating UI issues into manageable jobs.
For backend or system-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep control above jogging procedures and memory management. Understanding these instruments may have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with version Command systems like Git to know code historical past, uncover the precise instant bugs were being introduced, and isolate problematic adjustments.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about producing an personal familiarity with your progress ecosystem so that when issues arise, you’re not dropped in the dead of night. The higher you recognize your instruments, the more time it is possible to expend resolving the particular dilemma in lieu of fumbling by the method.
Reproduce the challenge
One of the more significant — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Just before leaping in to the code or creating guesses, builders will need to make a constant environment or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, generally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as you can. Ask thoughts like: What steps led to The difficulty? Which surroundings was it in — development, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it gets to isolate the exact ailments underneath which the bug occurs.
When you finally’ve collected plenty of details, seek to recreate the challenge in your local ecosystem. This could signify inputting exactly the same details, simulating comparable person interactions, or mimicking technique states. If The difficulty appears intermittently, take into consideration creating automatic checks that replicate the edge scenarios or state transitions included. These checks not just enable expose the problem but in addition protect against regressions Sooner or later.
Sometimes, the issue could possibly be ecosystem-particular — it would transpire only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for tolerance, observation, along with a methodical method. But after you can persistently recreate the bug, you happen to be now midway to correcting it. With a reproducible scenario, You can utilize your debugging equipment far more proficiently, take a look at opportunity fixes properly, and talk much more clearly together with your group or customers. It turns an abstract criticism into a concrete challenge — and that’s exactly where developers prosper.
Examine and Fully grasp the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. As an alternative to viewing them as aggravating interruptions, developers must discover to take care of mistake messages as direct communications in the system. They normally inform you what precisely took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Start by looking at the information thoroughly and in full. Lots of developers, especially when underneath time stress, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs might lie the correct root cause. Don’t just copy and paste mistake messages into search engines like google and yahoo — go through and understand them 1st.
Break the error down into areas. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line range? What module or perform brought on it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also beneficial to be familiar with the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can greatly accelerate your debugging system.
Some mistakes are obscure or generic, As well as in These situations, it’s very important to examine the context where the error occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings both. These normally precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a more productive and assured developer.
Use Logging Sensibly
Logging is Probably the most effective equipment within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, helping you comprehend what’s happening under the hood without needing to pause execution or step in the code line by line.
A very good logging system starts off with realizing what to log and at what degree. Typical logging levels include DEBUG, Facts, Alert, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic details in the course of advancement, Information for general situations (like thriving start out-ups), WARN for possible concerns that don’t break the applying, Mistake for real problems, and Lethal if the program can’t continue.
Stay away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and decelerate your method. Focus on critical activities, point out adjustments, input/output values, and significant choice details Developers blog with your code.
Format your log messages Plainly and regularly. Contain context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
All through debugging, logs Enable you to keep track of how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in manufacturing environments where by stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, smart logging is about equilibrium and clarity. Having a well-imagined-out logging solution, you are able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex undertaking—it is a form of investigation. To proficiently identify and repair bugs, developers have to tactic the process like a detective solving a thriller. This frame of mind assists break down sophisticated troubles into workable sections and abide by clues logically to uncover the root cause.
Begin by gathering proof. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, gather as much appropriate data as you may devoid of leaping to conclusions. Use logs, examination situations, and consumer reviews to piece with each other a clear image of what’s taking place.
Subsequent, form hypotheses. Ask you: What can be producing this habits? Have any alterations not too long ago been created on the codebase? Has this concern occurred before less than very similar conditions? The aim is to slender down options and establish probable culprits.
Then, examination your theories systematically. Make an effort to recreate the problem inside of a controlled atmosphere. For those who suspect a certain perform or ingredient, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay back near focus to small information. Bugs frequently disguise inside the the very least anticipated sites—just like a lacking semicolon, an off-by-a single error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes could disguise the actual challenge, just for it to resurface later.
And finally, maintain notes on Anything you tried out and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for long run problems and support Other people fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical abilities, technique problems methodically, and develop into more effective at uncovering hidden difficulties in complex methods.
Publish Checks
Writing exams is among the simplest ways to help your debugging abilities and All round growth effectiveness. Assessments don't just help catch bugs early but also serve as a safety net that offers you confidence when creating modifications in your codebase. A properly-examined application is simpler to debug as it lets you pinpoint particularly wherever and when a problem occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can promptly expose no matter if a selected bit of logic is Doing work as predicted. Each time a check fails, you instantly know exactly where to look, significantly lessening enough time put in debugging. Device exams are Specifically helpful for catching regression bugs—issues that reappear just after Earlier getting set.
Next, combine integration exams and finish-to-close tests into your workflow. These enable be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in intricate programs with several factors or companies interacting. If some thing breaks, your checks can let you know which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to think critically regarding your code. To check a attribute properly, you may need to comprehend its inputs, envisioned outputs, and edge instances. This standard of comprehending Obviously prospects to higher code structure and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a strong starting point. After the take a look at fails consistently, you'll be able to deal with fixing the bug and enjoy your check move when The difficulty is settled. This technique makes certain that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable process—assisting you catch far more bugs, a lot quicker and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, seeking Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for far too very long, cognitive tiredness sets in. You would possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. On this state, your brain gets to be much less efficient at problem-resolving. A brief walk, a coffee crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your emphasis. Lots of builders report locating the root of a problem when they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, In particular for the duration of for a longer time debugging periods. Sitting down before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer attitude. You may quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you ahead of.
If you’re caught, a great general guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do some thing unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weak spot—it’s a smart system. It provides your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Each bug you come across is a lot more than simply a temporary setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful in case you go to the trouble to reflect and examine what went Mistaken.
Start out by inquiring you a few important concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater procedures like device screening, code testimonials, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Make more robust coding behaviors relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you could proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specifically potent. Whether it’s via a Slack information, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the same challenge boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as important portions of your advancement journey. After all, several of the best developers are not the ones who generate excellent code, but individuals that constantly study from their errors.
In the long run, Every bug you correct provides a different layer for your ability established. So upcoming time you squash a bug, take a second to replicate—you’ll occur away a smarter, additional able developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.